I set a textbox value from another textbox by jquery like this:
$(
'input[id="Name"]'
).live(
'change keyup'
,
function
() {
var
urlSegment = $(
'input[id="UrlSegment"]'
);
urlSegment.val(
"Test"
);
});
I think the problem lies along with ajax cause when changing to server binding the value is posted.
The form is generated in a popup by the grid.
How do I solve this?
Regards,
Mattias
12 Answers, 1 is accepted
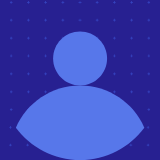
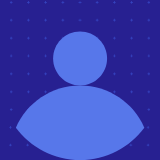
It seems to work by adding .change() to the textbox.
$(
'input[id="Name"]'
).live(
'change keyup'
,
function
() {
var
urlSegment = $(
'input[id="UrlSegment"]'
);
urlSegment.val(
"Test"
);
urlSegment.change();
});
/Mattias
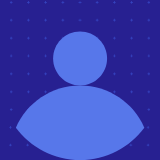

Hello,
When the jQuery val() method is used, the value of the input is visually changed. However, in order to have the same value applied in the model, you would have to manually trigger the change method so it would be indicated that the respective model's property has to be updated with the value of the input. Therefore, the provided suggestion above should be utilized:
$('input[id="Name"]').live('change keyup', function () {
var urlSegment = $('input[id="UrlSegment"]');
urlSegment.val("Test");
urlSegment.change();
});
Regards,
Tsvetomir
Progress Telerik

Good afternoon,
I am having this same issue, but using '.change()' isn't resolving the issue for me. I'm not sure what else to do.
I am auto filling a name and email textbox based on available users in the domain.
var Name = $('input[id="Name"]');
var Email = $('input[id="Email"]');
Name.bind("on keyup", function (e) {
var UN = $(this).val();
$.ajax({
cache: false,
type: "GET",
contentType: "application/json; charset=utf-8",
dataType: 'json',
url: url,
data: {"UserName": UN },
success: function (results) {
if (results) { alert("SUCCESS: " + results); }
var res = results.split(",");
var email = res[0];
var name = res[1];
if (name) {
Name.val(name);
Name.change();
Email.val(email);
Email.change();
}
},
error: function (xhr, ajaxOptions, thrownError) {
alert("AJAX error");
}
});
For some reason the name field gets posted successfully but email is always null even though I get the text I need in the textbox.
here is my grid
@(Html.Kendo().Grid<
vmPurchasingList
>()
.Name("PurchasingList")
.Columns(columns =>
{
columns.Bound(c => c.Name);
columns.Bound(c => c.Email);
columns.Bound(c => c.StepsString);
columns.ForeignKey(c => c.CostCenterId, (System.Collections.IEnumerable)ViewData["UsersCostCenters"], "Id","Name")
.Title("Cost Center");
columns.ForeignKey(c => c.StepsId, (System.Collections.IEnumerable)ViewData["Steps"], "Id", "Type")
.Hidden(true);
columns.ForeignKey(c => c.StepsHwyId, (System.Collections.IEnumerable)ViewData["StepsHwy"], "Id", "Type")
.Hidden(true);
columns.Command(command => { command.Edit(); }).Width(160);
})
.DataSource(dataSource => dataSource
.Ajax()
.PageSize(20)
.Events(events => {
events.Error("error_handler");
events.Sync("reload");
})
.Model(model =>
{
model.Id(c => c.Id);
model.Field(c => c.Id).Editable(false);
model.Field(c => c.Name);
model.Field(c => c.Email);
model.Field(c => c.CostCenterId).DefaultValue(1);
model.Field(c => c.StepsId).DefaultValue(0);
model.Field(c => c.StepsHwyId).DefaultValue(0);
})
.Create(create => create.Action("Create", "Home"))
.Read(r => r.Action("Read", "Home"))
.Update(update => update.Action("Update", "Home"))
)
.ToolBar(toolbar => toolbar.Create())
.Editable(editable => editable.Mode(GridEditMode.PopUp))
.Events(e => e.Edit("onEdit"))
.HtmlAttributes(new { style = "height:700px;" })
)
I am also having the same issue with the drop down lists when I attempt to 'clear' a users selection based on another drop down list.
Hi Luke,
As an alternative to triggering the change manually, you could set the value for the fields directly in the model and the binder should show the values in the textbox elements automatically:
var model = $("#grid").getKendoGrid().editable.options.model;
model.set("Name", name);
model.set("Email", email);
Regards,
Tsvetomir
Progress Telerik
Love the Telerik and Kendo UI products and believe more people should try them? Invite a fellow developer to become a Progress customer and each of you can get a $50 Amazon gift voucher.

Thank you, that did the trick. I implemented my code above inside the onEdit(event) call for my popup editing so my code was slightly different. I needed to update the model and the text of the textbox.
function onEdit(event) {
var model = event.model;
var Name = $('input[id="Name"]');
var Email = $('input[id="Email"]');
Name.bind("on keyup", function (e) {
var UN = $(this).val();
$.ajax({
cache: false,
type: "GET",
contentType: "application/json; charset=utf-8",
dataType: 'json',
url: autoFillUrl,
data: {"UserName": UN },
success: function (results) {
if (results) { alert("SUCCESS: " + results); }
var res = results.split(",");
var email = res[0];
var name = res[1];
if (name) {
Name.val(name);
Email.val(email);
model.Name = name;
model.Email = email;
}
},
error: function (xhr, ajaxOptions, thrownError) {
alert("AJAX error");
}
});
});
}
Hi Luke,
The set() method exposed by the observable objects is supposed to be updating the inputs automatically. Therefore, you would not have to manually fill the input as the binder will be automatically triggered.
https://docs.telerik.com/kendo-ui/api/javascript/data/observableobject/methods/set
Kind regards,
Tsvetomir
Progress Telerik
Virtual Classroom, the free self-paced technical training that gets you up to speed with Telerik and Kendo UI products quickly just got a fresh new look + new and improved content including a brand new Blazor course! Check it out at https://learn.telerik.com/.


var model = $(
"#grid"
).getKendoGrid().editable.options.model;
What is this sorcery?! I can't tell you how useful this little tidbit is...
Hi,
Whenever the Kendo UI Grid enters edit mode, a custom editable widget is dynamically created that has a reference to the currently edited item. An alternative to this would be to pass the TR element to the dataItem() method of the Kendo UI Grid:
https://docs.telerik.com/kendo-ui/api/javascript/ui/grid/methods/dataitem
Regards,
Tsvetomir
Progress Telerik
Love the Telerik and Kendo UI products and believe more people should try them? Invite a fellow developer to become a Progress customer and each of you can get a $50 Amazon gift voucher.