When setting the new CheckBoxes property to true on the RadComboBox, is it possible to customize the displayed text when items are checked?
Assume the following RadComboBox:
<
telerik:RadComboBox
ID
=
"rcbBlah"
runat
=
"server"
CheckBoxes
=
"true"
Width
=
"600px"
OnClientItemChecked
=
"OnClientItemChecked"
ChangeTextOnKeyBoardNavigation
=
"false"
>
<
Items
>
<
telerik:RadComboBoxItem
Text
=
"All"
Value
=
"-2"
/>
<
telerik:RadComboBoxItem
Text
=
"Not Specified"
Value
=
"-1"
Checked
=
"true"
/>
<
telerik:RadComboBoxItem
Text
=
"Apple"
Value
=
"1"
/>
<
telerik:RadComboBoxItem
Text
=
"Carrot"
Value
=
"2"
/>
<
telerik:RadComboBoxItem
Text
=
"Peach"
Value
=
"3"
/>
<
telerik:RadComboBoxItem
Text
=
"Pear"
Value
=
"4"
/>
<
telerik:RadComboBoxItem
Text
=
"Tomato"
Value
=
"5"
/>
<
telerik:RadComboBoxItem
Text
=
"Zucchini"
Value
=
"6"
/>
</
Items
>
</
telerik:RadComboBox
>
If the user selects Carrot and Tomato, our business users would prefer that the Text displayed be "Carrot,Tomato", not "Carrot, Tomato". Similarly, a business rule is that if we check the "All" item, we wrote JavaScript to check all of the items automatically. In this scenario, they want the Text displayed to be "All", not "Apple, Carrot, Peach, Pear, Tomato, Zucchini".
I tried catching the OnClientItemChecked event and doing the following, but that didn't do anything. It appears that the text property is readonly when checkboxes are utilized.
function
OnClientItemChecked(radComboBox, eventArgs) {
radComboBox.set_text(
'custom text for testing'
);
}
PS: Setting AllowCustomText doesn't work.
Thanks!!
Thad
14 Answers, 1 is accepted
I understand your point.
It makes sense to set the RadComboBox text to some general phrase (like "All") or to display the count of the checked items in control input, in case all (or large number of) RadComboBox items are selected.
Thank you for your suggestion, we will consider it for future releases.
As a token of our gratitude for your involvement we have updated your Telerik points.
Kalina
the Telerik team
Register for the Q2 2011 What's New Webinar Week. Mark your calendar for the week starting July 18th and book your seat for a walk through of all the exciting stuff we will ship with the new release!

I look forward to seeing this feature added in future releases.
-Thad
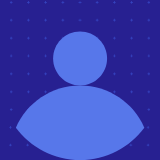
I am working on project and i am looking for the "Select All" functionality also. Would you be up for sharing your java function you created to do this?

function
OnClientItemChecked(radComboBox, eventArgs) {
var
currentItem = eventArgs.get_item();
var
isChecking = currentItem.get_checked();
var
allItems = radComboBox.get_items();
var
allItem = radComboBox.findItemByText(
'All'
);
var
nsItem = radComboBox.findItemByText(
'Not Specified'
);
var
anyChecked =
null
;
// Clicking on 'All' should result in all items other than 'All' and 'Not Specified' being checked.
// The 'All' checkbox is left unchecked because we don't want 'All' to appear in the text at the top.
if
(currentItem == allItem) {
allItems.forEach(
function
(item) {
if
(item == nsItem) {
item.set_checked(!isChecking);
}
else
if
(item == allItem) {
item.set_checked(!isChecking);
}
else
{
item.set_checked(isChecking);
}
});
}
// Clicking on 'Not Specified' should cause only the 'Not Specified' item to be checked.
// You cannot uncheck the 'Not Specified' checkbox except by checking other items.
else
if
(currentItem == nsItem) {
if
(isChecking) {
allItems.forEach(
function
(item) {
if
(item != nsItem) {
item.set_checked(
false
);
}
});
}
else
{
if
(nsItem) { nsItem.set_checked(
true
); }
}
}
// Clicking on a normal row should cause 'All' to be unchecked. If you are unchecking
// the last checked item, then the 'Not Specified' item should be checked, too.
else
{
var
anyChecked = isChecking;
if
(isChecking) {
if
(allItem) { allItem.set_checked(
false
); }
// Uncheck 'All'
if
(nsItem) { nsItem.set_checked(
false
); }
// Uncheck 'Not Specified'
}
else
{
if
(allItem) { allItem.set_checked(
false
); }
// Uncheck 'All'
allItems.forEach(
function
(item) {
if
((currentItem.get_text() != item.get_text()) && item.get_checked()) {
anyChecked =
true
;
return
;
}
});
if
(!anyChecked) {
if
(nsItem) { nsItem.set_checked(
true
); }
}
}
}
}
Enjoy,
Thad
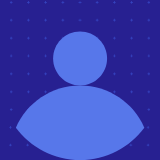
It works great.
I am trying to tweak it a bit what i want it to do is when i check the all it checks all the items (it does this currently)
At that time i also want it to set the text from "all" to "deselect all" (ie a toggle type action) and i have it were it will do set the text now but it gits rid of the check box in the combo when i reset the text. With out the check box there it wont refire event when trying to deselect all.
Any ideas on this??
Again thanks for the help guessing this will get used alot by folks till it is built in function.

This worked for me when I tried what you were suggesting... What is the code you were using?
// Put this with the other declarations
var
deselectItem = radComboBox.findItemByText(
'Deselect All'
);
// Put this at the end of the function
if
(currentItem == allItem) {
allItem.set_text(
'Deselect All'
);
}
if
(currentItem == deselectItem) {
deselectItem.set_text(
'All'
);
}
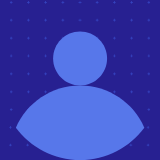
function OnClientItemChecked(radComboBox, eventArgs) {
var currentItem = eventArgs.get_item();
var isChecking = currentItem.get_checked();
var allItems = radComboBox.get_items();
var allItem = radComboBox.findItemByText(' ◄ Click to select all');
var nsItem = radComboBox.findItemByText(' ◄ Click to deselect all');
var anyChecked = null;
// Clicking on 'All' should result in all items other than 'All' and 'Not Specified' being checked.
// The 'All' checkbox is left unchecked because we don't want 'All' to appear in the text at the top.
if (currentItem == allItem) {
allItems.forEach(function (item) {
if (item == nsItem) {
item.set_checked(!isChecking);
}
else if (item == allItem) {
item.set_checked(!isChecking);
}
else {
item.set_checked(isChecking);
}
});
}
// Clicking on 'Deselect All' should cause only the 'Deselect All' item to be checked.
// You cannot uncheck the 'Deselect All' checkbox except by checking other items.
else if (currentItem == nsItem) {
if (isChecking) {
allItems.forEach(function (item) {
if (item != nsItem) {
item.set_checked(false);
}
});
}
else {
if (nsItem) { nsItem.set_checked(true); }
}
}
// Clicking on a normal row should cause 'All' to be unchecked. If you are unchecking
// the last checked item, then the 'Deselect All' item should be checked, too.
else {
var anyChecked = isChecking;
if (isChecking) {
if (allItem) {
allItem.set_checked(false);
} // Uncheck 'All'
if (nsItem) { nsItem.set_checked(false); } // Uncheck 'Deselect All'
}
else {
if (allItem) {
allItem.set_checked(false);
} // Uncheck 'All'
allItems.forEach(function (item) {
if ((currentItem.get_text() != item.get_text()) && item.get_checked()) {
anyChecked = true;
return;
}
});
if (!anyChecked) {
if (nsItem) { nsItem.set_checked(true); }
}
}
}
if (currentItem == allItem) {
allItem.set_text(' ◄ Click to deselect all');
}
if (currentItem == nsItem) {
nsItem.set_text(' ◄ Click to select all');
}
}

I am able to recreate your scenario today. Not sure why it worked fine for me yesterday, but will see if I can find the reason for this.
Thad

There appears to be a bug in the set_text method of the RadComboBoxItem.
Here is the code:
function
(e) {
var
c =
this
.get_element();
var
d =
this
.get_textElement();
if
(c && c.className.indexOf(
"rcbTemplate"
) == -1 && d) {
d.innerHTML = e;
}
this
._text = e;
this
._properties.setValue(
"text"
, e,
true
);
}
Note that it is setting d.innerHTML to the changed text, but when checkboxes are on, innerHTML is
<
li
class
=
"rcbItem "
>
<
input
type
=
"checkbox"
class
=
"rcbCheckBox"
>
" ◄ Click to select all"
</
li
>
Instead, use this function instead of Telerik's set_text method
function
set_text(item, newText) {
var
innerHTML = item.get_textElement().innerHTML;
item.get_textElement().innerHTML = innerHTML.substring(0, innerHTML.length - item.get_text().length) + newText;
item._text = newText;
item._properties.setValue(
"text"
, newText,
true
);
}
Your code will be:
set_text(allItem,
' ◄ Click to deselect all'
)
I'm sure there is prettier way of doing it, but this is all I have time to do right now. I'm sure it'll bother me enough to fix it up later.
Thad
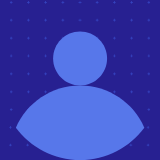
Thanks for the time spent. Works like i want it to now.
This is exactly why we went with telerik. The community and the support from the telerik guys.
I am sure the guys will find the issue and get it fixed up.
Again thanks for your time.
We have already planned to implement the “Check/Uncheck” logic in RadComboBox CheckBoxes feature for some of the next releases.
Regarding the bug in set_text() client-side method - we are aware of it, and we will schedule it for fixing.
Thank you for taking your time to research this - please find your Telerik points updated.
Best wishes,
Kalina
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
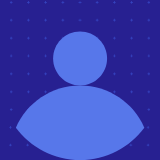
I am struck at a place where I need to set the RadComboBox text area to "Some Text", but even after setting the value I am not able see the value and it is displaying its Default value.
Is this a bug or am I missing any thing.
------------------------------------------------------
<telerik:RadComboBox ID="RadComboBox2" Label="Groups:" Filter="None" runat="server" MarkFirstMatch="true"
EmptyMessage="Select Groups" DropDownWidth="400px" HighlightTemplatedItems="true" ChangeTextOnKeyBoardNavigation="false"
OnClientItemChecked="OnClientItemCheckedHandler" TabIndex="3" AllowCustomText="true" CheckBoxes="true" EnableCheckAllItemsCheckBox="true"
Text="Select Groups" DataValueField="ID" DataTextField="Email">
</telerik:RadComboBox>
------------------------------------------------------
On OnClientItemCheckedHandler I am trying to set the comboBox.set_text(text).
Please help.
Regards,
M. Anil Kumar
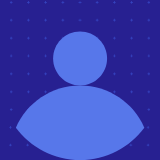
I am struck at a place where I need to set the RadComboBox text area to "Some Text", but even after setting the value I am not able see the value and it is displaying its Default value.
Is this a bug or am I missing any thing.
------------------------------------------------------
<telerik:RadComboBox ID="RadComboBox2" Label="Groups:" Filter="None" runat="server" MarkFirstMatch="true"
EmptyMessage="Select Groups" DropDownWidth="400px" HighlightTemplatedItems="true" ChangeTextOnKeyBoardNavigation="false"
OnClientItemChecked="OnClientItemCheckedHandler" TabIndex="3" AllowCustomText="true" CheckBoxes="true" EnableCheckAllItemsCheckBox="true"
Text="Select Groups" DataValueField="ID" DataTextField="Email">
</telerik:RadComboBox>
------------------------------------------------------
On OnClientItemCheckedHandler I am trying to set the comboBox.set_text(text).
Please help.
Regards,
M. Anil Kumar
I am afraid that setting the RadComboBox text is not supported when the CheckBoxes feature is enabled.
When a checkbox is checked - the text of the checked item automatically is displayed in the RadComboBox input - this behaviour is by design and is correct. That is why you are not able to set the text in the input to a custom text.
All the best,
Kalina
the Telerik team