16 Answers, 1 is accepted
Yes it is possible, you could handle the OnCalendarViewChanged event of the calendar and initiate Ajax request manually.
You will see an example in the code-snippets below.
Aspx:
<
telerik:RadScriptManager
ID
=
"RadScriptManager1"
runat
=
"server"
>
</
telerik:RadScriptManager
>
<
telerik:RadCodeBlock
ID
=
"RadCodeBlock1"
runat
=
"server"
>
<
script
type
=
"text/javascript"
>
function ViewChanged(sender, eventArgs) {
var step = eventArgs.get_step();
var ajaxManager = $find("<%= RadAjaxManager1.ClientID %>");
if (step) {
ajaxManager.ajaxRequest(step);
}
}
</
script
>
</
telerik:RadCodeBlock
>
<
div
>
<
telerik:RadAjaxManager
runat
=
"server"
ID
=
"RadAjaxManager1"
OnAjaxRequest
=
"RadAjaxManager1_AjaxRequest"
>
<
AjaxSettings
>
<
telerik:AjaxSetting
AjaxControlID
=
"RadAjaxManager1"
>
<
UpdatedControls
>
<
telerik:AjaxUpdatedControl
ControlID
=
"Label1"
/>
</
UpdatedControls
>
</
telerik:AjaxSetting
>
</
AjaxSettings
>
</
telerik:RadAjaxManager
>
<
asp:Label
runat
=
"server"
ID
=
"Label1"
Text
=
"no Ajax has performed yet"
></
asp:Label
>
<
telerik:RadDatePicker
ID
=
"RadDatePicker1"
runat
=
"server"
>
<
Calendar
>
<
ClientEvents
OnCalendarViewChanged
=
"ViewChanged"
/>
</
Calendar
>
</
telerik:RadDatePicker
>
C#:
protected
void
RadAjaxManager1_AjaxRequest(
object
sender, AjaxRequestEventArgs e)
{
int
step =
int
.Parse(e.Argument);
string
msg =
"The calendar moved "
;
if
(step < 0)
{
msg = msg +
"back "
;
step = -step;
}
else
{
msg = msg +
"forward "
;
}
msg = msg + step +
" views."
;
Label1.Text =
"Ajax callback performed at: "
+ DateTime.Now.ToString() +
" ; "
+ msg;
}
I hope this helps.
Best wishes,
Vasil
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
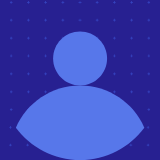
<script type=
"text/javascript"
>
function
Popup()
{
var
datePicker = $find(
"<%= rdpDate.ClientID %>"
);
datePicker.showPopup();
return
false
;
}
function
ViewChanged(sender, eventArgs) {
var
step = eventArgs.get_step();
var
ajaxManager = $find(
"<%= RadAjaxManager1.ClientID %>"
);
if
(step) {
ajaxManager.ajaxRequest(step);
}
}
</script>
<
telerik:RadDatePicker
ID
=
"rdpDate"
runat
=
"server"
DatePopupButton-Visible
=
"false"
OnSelectedDateChanged
=
"rdpDate_SelectedDateChanged"
AutoPostBack
=
"true"
>
<
DateInput
ID
=
"dateInput"
runat
=
"server"
CssClass
=
"HiddenTextBox"
/>
<
Calendar
>
<
ClientEvents
OnCalendarViewChanged
=
"ViewChanged"
/>
</
Calendar
>
</
telerik:RadDatePicker
>
John
I tested here the code using you Popup function to show the calendar and it still works fine. I am attaching the page that I used.
Could you explain in more details what is conflicting in your case? Do you get a JavaScript error or it is another problem?
All the best,
Vasil
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
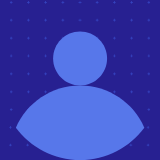
I believe the RadScriptManager trigger was causing the RadCalender to close itself. I've removed it, however, the calendar does not refresh to show the changes.
I am trying to get the functionality where the user changes the month on the calendar. This causes a postback to the server to get the list of special dates for that month from the database, and then highlight them back to the user.
I've tried the 2 approaches, but both are not as desired:
1)
<
telerik:RadAjaxManager
runat
=
"server"
ID
=
"RadAjaxManager1"
OnAjaxRequest
=
"RadAjaxManager1_AjaxRequest"
>
<
AjaxSettings
>
<
telerik:AjaxSetting
AjaxControlID
=
"RadAjaxManager1"
>
<
UpdatedControls
>
<
telerik:AjaxUpdatedControl
ControlID
=
"rdpDate"
/>
</
UpdatedControls
>
</
telerik:AjaxSetting
>
</
AjaxSettings
>
</
telerik:RadAjaxManager
>
This causes the calendar popup to close after clicking the next/previous month buttons.
2)
<
telerik:RadAjaxManager
runat
=
"server"
ID
=
"RadAjaxManager1"
OnAjaxRequest
=
"RadAjaxManager1_AjaxRequest"
>
</
telerik:RadAjaxManager
>
This causes the calendar not to refresh to display the highlighted dates.
My javascript and RadDatePicker tags are the same as above.
Thanks
John
Making rdpDate to update itself using the ajax settings should not cause it to close automatically. There could be an JavaScript error in your code that causes the popup to close.
Could you write us the code that you use for adding the special days?
For example I am using the code-below for dynamically adding the current selected day as special and it is working when calling it on page load.
protected
void
Page_Load(
object
sender, EventArgs e)
{
RadCalendar rC = rdpDate.Calendar;
RadCalendarDay rcD =
new
RadCalendarDay(rC);
if
(rdpDate.SelectedDate !=
null
)
{
rcD.Date = (DateTime)rdpDate.SelectedDate;
rcD.TemplateID =
"DateTemplate"
;
}
rC.SpecialDays.Add(rcD);
}
Best wishes,
Vasil
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
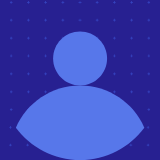
Here is my code for adding the special days. When the user clicks the next/previous month buttons on the calendar, it triggers the RadAjaxManager, which calls RadAjaxManager1_AjaxRequest with the focus date as the argument. I am attaching all of my code for completeness sakes.
<script type=
"text/javascript"
>
function
Popup()
{
var
datePicker = $find(
"<%= rdpDate.ClientID %>"
);
datePicker.showPopup();
return
false
;
}
function
ViewChanged(sender, eventArgs) {
var
month = sender.get_focusedDate();
var
ajaxManager = $find(
"<%= RadAjaxManager1.ClientID %>"
);
if
(month) {
ajaxManager.ajaxRequest(month);
}
}
</script>
<
telerik:RadAjaxManager
runat
=
"server"
ID
=
"RadAjaxManager1"
OnAjaxRequest
=
"RadAjaxManager1_AjaxRequest"
>
<
AjaxSettings
>
<
telerik:AjaxSetting
AjaxControlID
=
"RadAjaxManager1"
>
<
UpdatedControls
>
<
telerik:AjaxUpdatedControl
ControlID
=
"rdpDate"
/>
</
UpdatedControls
>
</
telerik:AjaxSetting
>
</
AjaxSettings
>
</
telerik:RadAjaxManager
>
<
asp:LinkButton
CssClass
=
"date"
runat
=
"server"
ID
=
"lbEdit"
OnClientClick
=
"return Popup();"
>
<
div
style
=
"position: relative;"
class
=
"calender"
>
<
telerik:RadDatePicker
Style
=
"display: block; height: 0px; top: 25px; position: absolute;"
ID
=
"rdpDate"
runat
=
"server"
DatePopupButton-Visible
=
"false"
OnSelectedDateChanged
=
"rdpDate_SelectedDateChanged"
AutoPostBack
=
"true"
>
<
DateInput
ID
=
"dateInput"
runat
=
"server"
CssClass
=
"HiddenTextBox"
/>
<
Calendar
ID
=
"calendar"
runat
=
"server"
>
<
ClientEvents
OnCalendarViewChanged
=
"ViewChanged"
/>
</
Calendar
>
</
telerik:RadDatePicker
>
</
div
>
<
i
class
=
"day"
>
<
asp:Literal
ID
=
"litDay"
runat
=
"server"
></
asp:Literal
></
i
> <
i
class
=
"month"
>
<
asp:Literal
ID
=
"litMonth"
runat
=
"server"
></
asp:Literal
></
i
> <
i
class
=
"dayno"
>
<
asp:Literal
ID
=
"litDate"
runat
=
"server"
></
asp:Literal
></
i
> <
b
>
<
asp:Literal
runat
=
"server"
Text
=
"edit"
></
asp:Literal
></
b
>
</
asp:LinkButton
>
protected
void
rdpDate_SelectedDateChanged(
object
sender, EventArgs e)
{
DateTime? _date = ((RadDatePicker)sender).SelectedDate;
litDate.Text = GetDate().ToString(
"dd"
);
litDay.Text = GetDate().ToString(
"ddd"
);
litMonth.Text = GetDate().ToString(
"MMM"
);
StringBuilder _sb =
new
StringBuilder();
for
(
int
i = 0; i < Request.QueryString.Count; i++)
{
if
(Request.QueryString.Keys[i] !=
"appDate"
)
{
_sb.Append(
"&"
).Append(Request.QueryString.Keys[i]).Append(
"="
).Append(Request.QueryString[i]);
}
}
Response.Redirect(Request.RawUrl.Split(
'?'
)[0] +
"?appDate="
+ SQS[
"appDate"
] + _sb.ToString());
}
protected
void
RadAjaxManager1_AjaxRequest(
object
sender, AjaxRequestEventArgs e)
{
string
[] _args = e.Argument.Split(
','
); ;
LoadCalendarDates(
int
.Parse(_args[1]),
int
.Parse(_args[0]));
}
private
void
LoadCalendarDates(
int
month,
int
year)
{
DataTable _dt = CDay.GetCalendarDates(WSession.user.user_id, month, year);
for
(
int
i = 0; i < _dt.Rows.Count; i++)
{
RadCalendarDay _day =
new
RadCalendarDay();
_day.Date = (DateTime)_dt.Rows[i][0];
_day.ItemStyle.BackColor = System.Drawing.Color.Orange;
rdpDate.Calendar.SpecialDays.Add(_day);
}
}
Thanks
John
As I see you are adding the special days properly, however the calendar stays hidden after you do it. To avoid this you can show it again after the callback. Here is an example code that you could use.
JavaScript:
var
oldMonthAsString =
""
;
function
Popup() {
var
datePicker = $find(
"<%= rdpDate.ClientID %>"
);
datePicker.showPopup();
}
function
ViewChanged(sender, eventArgs) {
var
month = sender.get_focusedDate();
var
ajaxManager = $find(
"<%= RadAjaxManager1.ClientID %>"
);
if
(month) {
var
monthAsString = month.toString();
if
(monthAsString != oldMonthAsString) {
oldMonthAsString = monthAsString;
ajaxManager.ajaxRequest(month);
}
}
}
protected
void
RadAjaxManager1_AjaxRequest(
object
sender, AjaxRequestEventArgs e)
{
string
[] _args = e.Argument.Split(
','
);
RadAjaxManager1.ResponseScripts.Add(
"Popup()"
);
LoadCalendarDates(
int
.Parse(_args[1]),
int
.Parse(_args[0]));
rdpDate.FocusedDate =
new
DateTime(
int
.Parse(_args[0]),
int
.Parse(_args[1]),
int
.Parse(_args[2]));
}
I hope this helps.
Best wishes,
Vasil
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
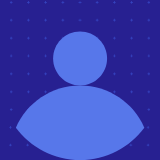
The calendar repops up, however, the focus date is not sticking when it is being set in RadAjaxManager1_AjaxRequest. It always shows the month with the selected date. I've wrapped the whole page inside a RadAjaxPanel.
John
This happens because the picker automatically set focus on the selected date when opening the calendar. You can handle ViewChanging and make a check if calendar is showing after clicking the show button, or after the ajax call. If the changing is caused automatically after the show/hide button click, you can cancel the event. Check this help topic for more information:
http://www.telerik.com/help/aspnet-ajax/calendar-on-calendar-view-changing.html
Regards,
Vasil
the Telerik team
Browse the vast support resources we have to jump start your development with RadControls for ASP.NET AJAX. See how to integrate our AJAX controls seamlessly in SharePoint 2007/2010 visiting our common SharePoint portal.
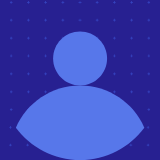
<
telerik:RadAjaxManagerProxy
runat
=
"server"
>
<
AjaxSettings
>
<
telerik:AjaxSetting
AjaxControlID
=
"RadAjaxManager"
>
<
UpdatedControls
>
<
telerik:AjaxUpdatedControl
ControlID
=
"calDate"
/>
</
UpdatedControls
>
</
telerik:AjaxSetting
>
</
AjaxSettings
>
</
telerik:RadAjaxManagerProxy
>
<
telerik:RadCodeBlock
ID
=
"RadCodeBlock1"
runat
=
"server"
>
<
script
type
=
"text/javascript"
>
function ViewChanged(sender, eventArgs) {
var step = eventArgs.get_step();
var ajaxManager = $find("<%= Master.FindControl("RadAjaxManager").ClientID %>");
if (step) {
ajaxManager.ajaxRequest(step);
}
}
</
script
>
</
telerik:RadCodeBlock
>
protected
void
Page_Load(
object
sender, EventArgs e)
{
rdpDate.Calendar.DayRender += Calendar_DayRender;
}
void
Calendar_DayRender(
object
sender, Telerik.Web.UI.Calendar.DayRenderEventArgs e)
{
// This stays on the same month
}
Check this Code Library, I think it is the same as what you are trying to achieve:
The difference is that the calendar is in multi month view. But the actual problem that is resolved is that it requires postback during the changing the views. The same code is valid for single view calendar with AutoPostBack set to true.
http://www.telerik.com/community/code-library/aspnet-ajax/calendar/how-to-use-raddatepicker-with-multimonthview-calendar.aspx
All the best,
Vasil
the Telerik team
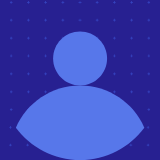
For me, the DayRender should work for whatever the month the user navigates to and shouldn't require manual AJAX firing etc.
We are thinking of making the DatePicker to handle AutoPostBack internally, in order to fire the server event, and to be able to open the calendar without firing it's ViewChanging after the page loads the second time. It will be possible by using a new property that will determinate if the calendar's view should re-render client side or server side. We will try to schedule it for our future release.
Kind regards,
Vasil
the Telerik team
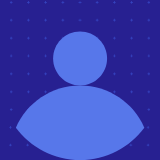
I tried getting this to work again, but with no luck.
When you navigate to the previous month, I want to do a server-side call (updating the special days), then repaint the displayed view. Unfortunately what happens is:
- If you have the Date Picker registered for Ajax (ram.AjaxSettings.AddAjaxSetting(ram, rdpDate);), the special days work, but the calendar pop-up doesn't work properly (it opens/closes erratically).
- If you don't have it registered for Ajax, the special days don't work.
I even tried reversing the problem by using a endRequestHandler with a RegisterDataItem on the RadScriptManager, but because I can't set Special Days on the Client Side, I can't think of any way of repainting the displayed calendar pop-up's month.
So yes, I'll await your changes - in the meantime, I'll have to populate all possible special days. I don't think this will scale well however.
The improvement is already scheduled for our next release, and we are working on it.
About the current workaround, please check the code library, it is working project. Al you have to do is to add your special days as you would do it for normal calendar.
All the best,
Vasil
the Telerik team
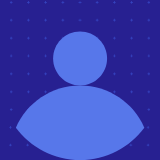
It works at the start when I navigate between months, however when I add a Special Day, navigate away and then navigate back, the Css Style is somehow lost (the code-behind confirms it should be a different colour). I am clearing the special days collection first of course, but not having much luck with it.
Anyway, I've created a support ticket.