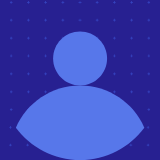
protected
override
void
SetupFilterControls(TableCell cell)
{
RadComboBox rcBox =
new
RadComboBox();
rcBox.ID =
this
.GetType().Name +
"ComboBox"
;
rcBox.AutoPostBack =
true
;
rcBox.DataTextField =
this
.DataField;
rcBox.DataValueField =
this
.DataField;
rcBox.SelectedIndexChanged += rcBox_SelectedIndexChanged;
rcBox.Items.Add(
new
RadComboBoxItem(
"Show All"
,
""
));
rcBox.DataSource = DataSource;
//<-------- I need to change this DataSource depending on what data is in my RadGrid.
//How can I pass a value here from my aspx page
rcBox.AppendDataBoundItems =
true
;
cell.Controls.Add(rcBox);
}
7 Answers, 1 is accepted
You have access to the MasterTableView in the SetupFilterControls method from where you can access the datakey names and basically the value of any cell in the grid:
protected
override
void
SetupFilterControls(System.Web.UI.WebControls.TableCell cell)
{
var masterTableView =
this
.Owner;
masterTableView.DataKeyNames
masterTableView.DataKeyValues
}
Marin
the Telerik team
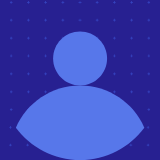
The value of a cell for a specific row and column can be accessed like this:
protected
override
void
SetupFilterControls(TableCell cell)
{
var masterTableView =
this
.Owner;
var value = masterTableView.Items[i][
this
].Text;
}
or if you have set its data field as a data key name, you can also access it like this:
var keyValue = masterTableView.DataKeyValues[i][
"DataKeyName"
];
Marin
the Telerik team
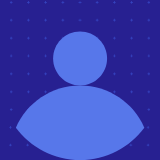
"Index was out of range. Must be non-negative and less than the size of the collection. Parameter name: index"
<
telerik:RadGrid
ID
=
"uxEmpGrid"
runat
=
"server"
AutoGenerateColumns
=
"false"
AllowSorting
=
"True"
GroupingEnabled
=
"true"
ShowGroupPanel
=
"true"
Skin
=
"Office2007"
AlternatingItemStyle-BackColor
=
"#EEEEEE"
GridLines
=
"None"
ShowFooter
=
"true"
AllowPaging
=
"true"
PageSize
=
"200"
AllowFilteringByColumn
=
"true"
>
<
GroupingSettings
ShowUnGroupButton
=
"true"
CaseSensitive
=
"false"
/>
<
ClientSettings
AllowDragToGroup
=
"true"
></
ClientSettings
>
<
MasterTableView
DataKeyNames
=
"DivisionID"
>
<
Columns
>
<
telerik:GridBoundColumn
DataField
=
"DivisionID"
HeaderText
=
"DivisionID"
Groupable
=
"false"
></
telerik:GridBoundColumn
>
<
telerik:GridBoundColumn
DataField
=
"SAPEmpNum"
HeaderText
=
"ID"
Groupable
=
"false"
></
telerik:GridBoundColumn
>
<
telerik:GridBoundColumn
DataField
=
"FullNameLastNameFirst"
HeaderText
=
"Team Member"
></
telerik:GridBoundColumn
>
<
nu:SAPPositionFilterColumn
DataField
=
"SAPPosition"
HeaderText
=
"Job Title"
UniqueName
=
"SAPPosition"
>
<
Itemtemplate
>
<%# Eval("SAPPosition") %>
</
Itemtemplate
>
</
nu:SAPPositionFilterColumn
>
<
nu:SAPCrewFilterColumn
DataField
=
"SAPCrew"
HeaderText
=
"Crew"
UniqueName
=
"SAPCrew"
>
<
Itemtemplate
>
<%# Eval("SAPCrew") %>
</
Itemtemplate
>
</
nu:SAPCrewFilterColumn
>
<
nu:SAPDepartmentFilterColumn
DataField
=
"SAPDepartment"
HeaderText
=
"Department"
UniqueName
=
"SAPDepartment"
>
<
Itemtemplate
>
<%# Eval("SAPDepartment") %>
</
Itemtemplate
>
</
nu:SAPDepartmentFilterColumn
>
<
nu:SAPSupervisorFilterColumn
DataField
=
"SAPSupervisorNameLastNameFirst"
HeaderText
=
"Supervisor"
UniqueName
=
"SAPSupervisorNameLastNameFirst"
>
<
Itemtemplate
>
<%# Eval("SAPSupervisorNameLastNameFirst") %>
</
Itemtemplate
>
</
nu:SAPSupervisorFilterColumn
>
</
Columns
>
</
MasterTableView
>
</
telerik:RadGrid
>
protected
override
void
SetupFilterControls(TableCell cell)
{
var masterTableView =
this
.Owner;
//Either one gives an error
this
.DivisionID = Convert.ToInt32(masterTableView.DataKeyValues[0][
"DivisionID"
]);
this
.DivisionID = Convert.ToInt32(masterTableView.Items[0][
this
].Text);
RadComboBox rcBox =
new
RadComboBox();
rcBox.ID =
this
.GetType().Name +
"ComboBox"
;
rcBox.AutoPostBack =
true
;
rcBox.DataTextField =
this
.DataField;
rcBox.DataValueField =
this
.DataField;
rcBox.SelectedIndexChanged += rcBox_SelectedIndexChanged;
rcBox.Items.Add(
new
RadComboBoxItem(
"Show All"
,
""
));
rcBox.DataSource = DataSource;
rcBox.AppendDataBoundItems =
true
;
cell.Controls.Add(rcBox);
}
Most likely the error is causes because the grid is not databound yet - and its column (and datakay values) still do not contain any data. Normally the grid first builds all the controls (filter controls, column editors etc.) and then populates them with data. So building a filter control that depends on the data of another column cannot be achieved in this way because the actual data that you need is not yet populated in the grid.
Another option is to add the filter control later in the page life cycle after the grid has been databound (but before it is rendered) and then access the necessary data. One such event is the ItemDataBound event. Of course you can also manually perform a query to the database and extract the necessary data if this is applicable in your case.
Marin
the Telerik team
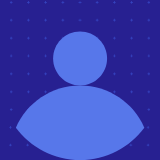
You could handle the DropDown SelectedIndexChanged event and manipulate the FilterExpression, similar to this:
<
telerik:RadComboBox
ID
=
"RadComboBox1"
runat
=
"server"
AutoPostBack
=
"true"
onselectedindexchanged
=
"RadComboBox1_SelectedIndexChanged"
>
<
Items
>
<
telerik:RadComboBoxItem
Text
=
"1"
Value
=
"1"
/>
<
telerik:RadComboBoxItem
Text
=
"2"
Value
=
"2"
/>
</
Items
>
</
telerik:RadComboBox
>
<
telerik:RadGrid
ID
=
"RadGrid1"
runat
=
"server"
AutoGenerateColumns
=
"false"
OnNeedDataSource
=
"RadGrid1_NeedDataSource"
AllowFilteringByColumn
=
"true"
EnableLinqExpressions
=
"false"
>
<
MasterTableView
>
<
Columns
>
<
telerik:GridBoundColumn
DataField
=
"ID1"
HeaderText
=
"ID1"
UniqueName
=
"ID1"
>
</
telerik:GridBoundColumn
>
<
telerik:GridBoundColumn
DataField
=
"ID2"
HeaderText
=
"ID2"
UniqueName
=
"ID2"
>
</
telerik:GridBoundColumn
>
<
telerik:GridBoundColumn
DataField
=
"ID3"
HeaderText
=
"ID3"
UniqueName
=
"ID3"
>
</
telerik:GridBoundColumn
>
<
telerik:GridEditCommandColumn
UniqueName
=
"EditColumn"
>
</
telerik:GridEditCommandColumn
>
</
Columns
>
</
MasterTableView
>
</
telerik:RadGrid
>
C#:
protected
void
RadGrid1_NeedDataSource(
object
sender, Telerik.Web.UI.GridNeedDataSourceEventArgs e)
{
dynamic data =
new
[] {
new
{ ID1 =
"1"
, ID2 = 11, ID3=111},
new
{ ID1 =
"2"
, ID2 = 22, ID3=222}
};
RadGrid1.DataSource = data;
}
protected
void
RadComboBox1_SelectedIndexChanged(
object
sender, Telerik.Web.UI.RadComboBoxSelectedIndexChangedEventArgs e)
{
RadGrid1.MasterTableView.FilterExpression =
"([ID1] LIKE \'%"
+ RadComboBox1.SelectedValue +
"%\') "
;
GridColumn column = RadGrid1.MasterTableView.GetColumnSafe(
"ID1"
);
column.CurrentFilterFunction = GridKnownFunction.Contains;
column.CurrentFilterValue = RadComboBox1.SelectedValue;
RadGrid1.MasterTableView.Rebind();
}
I hope this helps.
Greetings,
Maria Ilieva
the Telerik team