I have a Model with several nullable bool objects, which when I break point and check the values come back form the database correctly. However, when the page is loaded the value is displayed on the grid but when I use the Edit Popup, if the Value is Null, the correct drop down value "Not Set" is selected, but if there is a True/False value the selected value is blank, which will also key off the validation on submission.
Here is an example of the model.
public
class
CommitteeViewModel
{
[Required]
[ScaffoldColumn(
false
)]
public
int
CommitteeId {
get
;
set
; }
public
bool
? AllowUpdatesByOthers {
get
;
set
; }
}
And my View
<%=
Html.Telerik()
.Grid<
CommitteeViewModel
>()
.Name("Grid")
.DataKeys(k => k.Add(c => c.CommitteeId))
.ToolBar(commands => commands.Insert().ButtonType(GridButtonType.ImageAndText).ImageHtmlAttributes(new {style = "margin-left:0"}))
.DataBinding(databinding => databinding.Ajax()
.Select("_GetAllCommittees", "Committee")
.Insert("_CreateCommittee", "Committee")
.Update("_EditCommittee", "Committee")
.Delete("_DeleteCommittee", "Committee"))
.Columns(columns =>
{
columns.Bound(e => e.FullName);
columns.Bound(e => e.ShortName);
columns.Bound(e => e.AllowUpdatesByOthers);
columns.Command(commands =>
{
commands.Edit().ButtonType(GridButtonType.Image);
commands.Delete().ButtonType(GridButtonType.Image);
}).Width(70);
})
.Editable(editing => editing.Mode(GridEditMode.PopUp))
.Scrollable(scrolling => scrolling.Height(300))
.Filterable()
.Sortable()
.Pageable()
%>
Any Suggestions?
Thanks in advance.
-Karl
10 Answers, 1 is accepted
I was able to recreate the behavior you have described. However, I'm happy to inform you that we have managed to address it and I have attached an internal build in which this functionality should be supported.
Regards,Rosen
the Telerik team
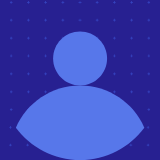
I have a batch editing grid which is bound in server mode and then updates in Ajax.
- When it binds I see the drop down with either True,Fale or Not Set
- When I click to edit I see that it becomes editable and I can change the dropdown.
- When I click away it reverts back to 'true' or 'false', not the original server bound Drop down.
My guess is this is a product of my Server/Ajax scenario.
Actually my issue is that I simply like to hide the Nullable aspect from the user and only show a CheckBox.
I tried in vain to create a ClientTemplate that would process a Nullable<bool> but could not get it to even render, I resorted to creating a bool wrapper property around my nullable bool property and binding to that. This is acceptable now but not going forward, is there a nugget of jquery template you can provide that can accept a nullable bool and present a check box in either checked/unchecked state?
PS. Is there any reason that Telerik does not default to using a checkbox for a bool in Ajax mode?
I also believe you could auto generate these templates in future versions, something like a set of optional Ajax client control Extensions e.g
public
static
GridBoundColumnBuilder<TModel> EditableBool<TModel, TValue>(
this
GridColumnFactory<TModel> columnFactory, Expression<Func<TModel, TValue>> expression)
where TModel :
class
{
var c = columnFactory.Editable(expression);
var template =
"<input type='checkbox' disabled='disabled' name='"
+ c.Column.Member +
"' <#= "
+
c.Column.Member +
" ? checked='checked' : '' #> />"
;
return
c.ClientTemplate(template);
}
Thanks.
As you may know our grid component uses the built-in MVC templating functionality, thus the display and editor templates will be used for presenting the property's values. Therefore, there are several way in order to change the editor for a particular property, for example you may change the type's default template or "attach" a custom template to a model's property through adding a UIHint attribute. More information on display and editor templates can be found in this help article.
Rosen
the Telerik team
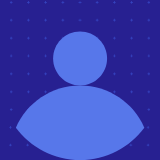
The issue is:
Batch editing grid server bound:
Bind Server: Cell contains disabled Checkbox (correct)
Click on cell to edit: Cell contains editable Checkbox (as per custom specified ClientTemplate)
Click away: Cell now displays 'true' (why not original Checkbox?)
How is it that clicking away generates a different control than you had renedered server side? I did not specify a UIHint so I assume the server rendering of the checkbox is a Telerik choice, if so surely your ajax Display rendering supports the same checkbox?
I also note that you have hidden on your Batch Editing Grid Controller code from your demo site, is this intentional?
This is a well known issue related with display templates. When using server binding the grid uses the display template which by default is a disabled checkbox. Batch editing however is not aware of this (it is a pure client-side feature). You need to specify a client-side template as mentioned in the troubleshooting help article.
I am not sure what you mean by hiding the grid controller. The controller and view are available in the code viewer.
Atanas Korchev
the Telerik team
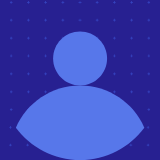
http://demos.telerik.com/aspnet-mvc/razor/grid/editingbatch
Then click the view tab.
Note: and you have also broken the Razor link again, this worked last week but now just defaults to ASPX.
I strongly believe that telerik need to fix the Ajax / Server template design, it is rally not clear even having read all the documentation I am still confused (check stack overflow) and to expect everyone to read the Troubleshooting guide just to be aware of extremely important information like this is unacceptable. Please promote this section to Documentation.
As I have suggested elsewhere you should provide a set of Ajax supporting columns that abstracts ClientTemplate = 'a load of horrible jquery that produces what teleriks own server controls produce' for developers. Why not follow MS lead of producing Jquery display and editor templates for Ajax, surely this is in the pipeline?
I just ran into another issue, how do I make an individual cell read only. I don't think this is possible!
I tried CellAction, check column and row set Template = '' but because you do not expose read only property and no way of setting the client template I cannot implement the required business logic! I now have consider completely redesigning the grid because or actually creating a custom build of Telerik.MVC.
Indeed there is something wrong in the online demo (the razor version). Until we fix it you can use the sample project which we ship with the Telerik installation.
I agree that there are some gotchas when dealing with templates. For starters we have 4 different kinds of templates - server, client, display and edit templates. Have in mind though that Telerik templates (server and client) were implemented earlier than display and editor templates (which were shipped in ASP.NET MVC 2). In a perfect world we would have only supported only display and editor templates. However it is not technically possible to support display and editor templates 100% on the client-side. The biggest obstacle is that display and editor templates are defined server side whereas client-side templates must be defined in either JavaScript or some templating language (such as jQuery templates).
"As I have suggested elsewhere you should provide a set of Ajax supporting columns that abstracts ClientTemplate = 'a load of horrible jquery that produces what teleriks own server controls produce' for developers. Why not follow MS lead of producing Jquery display and editor templates for Ajax, surely this is in the pipeline?"
Could you please clarify what you mean? I guess you want predefined set of columns but what that predefined set should be? Every developer has unique requirements and different needs. Also I am not aware of any MS development with regards to client-side display and edit templates. Let me know (e.g. post some links) if you know of something related.
Finally to make a column readonly use the ReadOnly method:
columns.Bound(p => p.ProductName).Width(210).ReadOnly();
Regards,
Atanas Korchev
the Telerik team
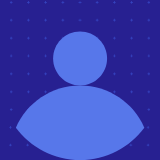
Let me clarify my points starting with the most imporant:
1. I would like a ReadOnly cell. Not column. I have 3 subtotals rows which are generated by a stored proc and as such it becomes very hard to use footer, so I thought great I will use Cell and RowAction to customise the look and feel. The problem is because you do not expose ClientTemplate in GridCell<T> I cannot in my Grid CellAction method set readonly based on BOTH row and column. This is a big problem which I cannot see an easy way around. I believe there should be a onBeginEdit client side event which allow me to preventDefault but this is not exposed. This is why I cannot put everything client side, I have already implemented in server side.
Could you please log a feature request for this?
2. To clarify my comment 'jquery that produces the same output as Telerik server...': What I am saying is if I write BoundColumn(c=>c.SomeBoolProperty) and I DO NOT specify a UIHint I should expect the Telerik code to produce a server generated control (in this case checkbox) that is consistent with the ajax or client side version (which it currently is not). You say that you could not know what control to use but actually you are already making that guess with the display templates (hence I get a checkbox without specifying a UIHint or any Display or Editor templates for bool) so why not implement a matching set of jquery ClientTemplates (as per your demo example) rather than putting this requirement on the developer to implement ClientTemplate = 'some jquery to produced editor'. Perhaps this is a feature request but I think it would make using your grid 100% more intuitive. On a different ticket I suggested releasing an AjaxExtensions class that would add ColumnBuilder overrides (something I have been doing over the weekend) grid.AjaxBool(c=>c.SomeBoolProperty) But point 3 perhaps is a different path for the same problem:
3. 'Why not follow MS lead of producing Jquery display and editor templates for Ajax, surely this is in the pipeline?' What I meant was why not implement a Jquery version of the MS MVC Editor and Display template system that mimic. So to generate the ClientTemplate Telerik code would look in a predefined folder perhaps ~/Views/ClientDisplayTemplates and~/Views/ClientEditorTemplates and look for a boolean.cshtml or a MyType.cshtml, this could lead to you adding overrides to your controls that would spit out Jquery compliant controls e.g NumericTextbox.RenderJquery(). This would suddenly remove the requirement to implement all my controls in both server side and jquery. Or better you could just add ClientTemplate property to all your MVC controls and let it generate the Jquery. Just some ideas.
Tom.
You can implement read only cells by subscribing to their "click" event and prevent DOM event bubling. That way the grid won't try to edit that cell. Here is some sample code which will disallow editing the first cell:
function
onDataBound() {
$(
this
).find(
"tbody > tr > td"
).click(
function
(e) {
if
(
this
.cellIndex === 0) {
e.stopPropagation();
}
});
}
Currently the grid is using Html.DisplayFor when rendering the grid from server side (server binding). We cannot be sure what ASP.NET MVC will output for a particular property type (int, bool etc) in order to provide its client-side counterpart. So far the boolean is the only thing which behaves funny (renders as disabled checkbox in ASP.NET MVC 2/3 and as a string in ASP.NET MVC 1) but things may change in the future.
I think that introducing yet another way to specify templates would be confusing. Users will be completely lost with two new ways to specify a template ("Should I use ClientTemplate, DisplayTemplate or ClientDisplayTemplate?"). And what happens if the developer must support both server and ajax binding in the same grid (we have such implementations)?
Currently we have specified that display templates are not supported in ajax binding. Users should use ClientTemplates with this regard.
We do our best to support server editor templates in ajax binding scenarios. There are some quirks (such as the fact that any server code defined is in the editor template is executed only once against dummy data in order to output the editor html) but it works in most cases. Even if something fails users can hook up to the OnEdit event and initialize the editor properly. Perhaps one day we would implement ClientEditTemplate similar to the ClientTemplate which does not rely on ASP.NET MVC editor templates and server code. Regards,
Atanas Korchev
the Telerik team
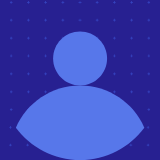
Well, I now see that you are calling Html.DisplayFor() and now that you have explained how you generate the Ajax editor using dummy data things are starting to make sense, hmmm.. ok I think this is crucial information, perhaps it is included in the documentation but I just didn't read it properly.
Thanks.