$.ajax({
url: "http://someserver:8554/search",
type: 'POST',
beforeSend: function(req) {
req.setRequestHeader('Authorization', auth);
},
success: function(data) {
log(data);
}
});
auth is a base64 encoded string that I create that contains the user and password.
Can this be done with the Kendo DataSource?
9 Answers, 1 is accepted
As you probably know, when remote requests are used, DataSource by default will use jQuery ajax to make those requests. Having this in mind you may use the transport's read options to pass additional parameters which will be then assign to the $.ajax. For example:
new
kendo.data.DataSource({
transport: {
read: {
url:
"http://someserver:8554/search"
,
type:
'POST'
,
beforeSend:
function
(req) {
req.setRequestHeader(
'Authorization'
, auth);
}
}
},
change:
function
() {
log(
this
.data());
}
}).read();
Regards,
Rosen
the Telerik team
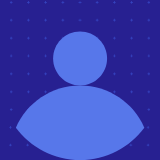
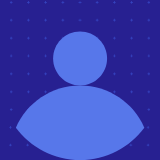
The Auth string is correct and works fine in "Advanced Rest Client" in my browser.
==================================================================
define(function () {
var dataSource = new kendo.data.DataSource({
transport: {
read: window.apiRoot + 'accounts',
type: "GET",
beforeSend: function (xhr) {
xhr.setRequestHeader('Authorization', window.auth);
}
}
});
return dataSource;
});
The Network tab shows a RED request, and when I look at it, the Authentication header is NOT there.
I'm going out of my mind in frustration - I've been at this for 2 hours.
I know the datasource works because when I populate it with dummy data I can use it - the problem HAS to be that the transport is not sending the header.
Any ideas?
The beforeSend option should be passed to transport.read, not to the transport directly:
var dataSource = new kendo.data.DataSource({
transport: {
read: {
url: window.apiRoot + 'accounts',
type: "GET",
beforeSend: function (xhr) {
xhr.setRequestHeader('Authorization', window.auth);
}
}
}
});
Regards,
Alex Gyoshev
Telerik
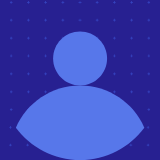
Hello Grahame,
I'm afraid that we do not have example of how to use JWT with UI for ASP.NET MVC. However, the approach for sending the auth token as a custom request header similar as describe in this issue can be used. Unfortunately, the current way of setting custom request headers via the wrappers is a bit cumbersome.
If you need further assistance please feel free to open a separate support request, as your question is not directly related to the one discussed in this thread.
Rosen
Telerik by Progress
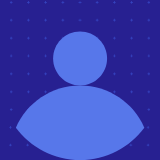
I've tried this every way I could think of... nothing seems to put the token in the request header. If I set the server to allow anonymous access then the method returns data as expected.
<div id="listView"></div>
<script type="text/x-kendo-tmpl" id="template">
<div class="BoxAthletes">
<h3>#:username#</h3>
</div>
</script>
<script>
var dataSource = new kendo.data.DataSource({
transport: {
read: {
url: "https://wodmodeapi.azurewebsites.net/api/v/p/athletes",
dataType: "jsonp",
//headers: { 'Authorization': 'Bearer 5bY8Ot8X-m4tYoF_rjVjFdYwtt_LQfzS6yqgy-c4jzUzDxwnajRKChNU7m_NxSbu4eaLQAoMzNc-lPBvIUWaZdIeACabECoK44QdU8fnlrJAkdBa9aQiCTUuxUhuAL1VWQv5T-OfALPGFA96E9Y0-ZBxwZlhawVHcWDRGP2378WzF3gX35L1c5d0k_5KegT97t7-_fOTakLWN4kCk6QwG_-gSCGoBTSjktl5tKGXXgE' }
beforeSend: function (req) {
req.setRequestHeader("Authorization", "Bearer 5bY8Ot8X-m4tYoF_rjVjFdYwtt_LQfzS6yqgy-c4jzUzDxwnajRKChNU7m_NxSbu4eaLQAoMzNc-lPBvIUWaZdIeACabECoK44QdU8fnlrJAkdBa9aQiCTUuxUhuAL1VWQv5T-OfALPGFA96E9Y0-ZBxwZlhawVHcWDRGP2378WzF3gX35L1c5d0k_5KegT97t7-_fOTakLWN4kCk6QwG_-gSCGoBTSjktl5tKGXXgE");
}
}
},
schema: {
model: {
id: "id",
fields: {
id: { type: "number" },
email: { type: "string" },
username: { type: "string" },
tagline: { type: "string", nullable: true },
url: { type: "string", nullable: true },
created_dt: { type: "date" },
edited_dt: { type: "date" },
birth_dt: { type: "date" },
crossfit_dt: { type: "date" },
gender: { type: "string" },
img_guid: { type: "string" },
score_privacy: { type: "string" },
banned_flag: { type: "number" }
}
}
}
});
$("#listView").kendoListView({
dataSource: dataSource,
pageable: true,
template: kendo.template($("#template").html())
});
</script>
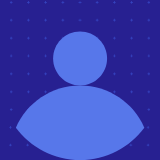
You can also check out the following documentation article on CORS data fetching for additional information:
Regards,
Dimitar
Progress Telerik