I have a grid very similar to the one in this example where I am using 3 different client templates to populate and bind 3 drop downs in my grid:
https://demos.telerik.com/aspnet-core/grid/editing-custom
My dropdowns are:
- Category
- Department
- Configuration
I have a business rule whereby if the user chooses one particular category then I need to set the Configuration drop down to it's first selection (which is 'NONE') and disable it so that the user is unable to change it. If the user chooses any other category, I need to re-enable that Configuration dropdown so that they can make a choice. How do I tackle this?
8 Answers, 1 is accepted
Hello Scott,
To achieve this functionality I would suggest subscribing to the Change event of the Category DropDownList and in the event handler conditionally set the Configuration DropDownList readonly:
function onChange(e) {
var dropDown = $("#Age").data('kendoDropDownList') // Age is the name of the broperty bound to the column
this.value() == "None" ? dropDown.readonly() : dropDown.readonly(false);
}
For your convenience, I am attaching a small project demonstrating this. Please note it is an MVC one but the implementation is valid for Core projects as well.
Let me know if you have any questions.
Regards,
Nikolay
Progress Telerik
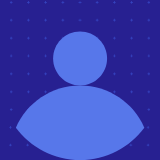
I don't get where you're getting 'Age' from, that's confusing...
I have 3 drop downs, Category, Department, Configuration. They are bound as columns in the Grid like this:
columns.Bound(e => e.Category).ClientTemplate("#=Category.CategoryName#").Title("Category");
columns.Bound(e => e.Department).ClientTemplate("#=Department.DepartmentName#").Title("Department");
columns.Bound(e => e.Configuration).ClientTemplate("#=Configuration.ConfigurationName#").Title("Configuration");
if I try to implement your onChange function event handler I can retrieve the Category drop down in the way that you specify but the Configuration drop down returns undefined. To Illustrate:
function onCategoryChange(e) {
var catDD = $("#Category").data("kendoDropDownList");
alert("cat is '" + catDD.value() + "'"); //displays correct value
var configDD = $("#Configuration").data("kendoDropDownList");
alert("config is '" + configDD.value() + "'"); //TypeError: configDD is undefined
}
oddly, if I add an event handler to the Change event of the Configuration drop down, it will print out 'Configuration' :
function onConfigurationChange(e) {
var id = this.element.attr("id");
alert("ID is '" + id + "'"); //displays Configuration
}
Its like it doesn't think the Configuration drop down exists unless you are interacting with it directly. Any idea what is going on here?
Hello Scott,
In the project, I shared the Age is actually the model property bound to the column with Title "Configuration" and so to access this DropDownList editor in the Change event of another one the model property name should be used:
columns.Bound(x => x.Age).Title("Configuration")
function onChange(e) {
var dropDown = $("#Age").data('kendoDropDownList') // Age is the name of the property bound to the column
...
Additionally, the selected DropDown value in the change event handler could be accessed via this.value() which will return the value already specified with DataValueField().
I have modified the project setting the Category (Age) column editor using a remote bindin. Please note that it is only for demonstrative purposes and therefore the data is not real-world relevant.
Regards,
Nikolay
Progress Telerik
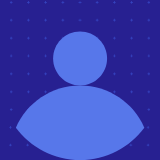
Nope,sorry it's gotta be something else. My code is EXACTLY like your example except I have ClientTemplate defined in my grid columnbound expressions
columns.Bound(e => e.Configuration).ClientTemplate("#=Configuration.ConfigurationName#").Title("Configuration");
and for some reason I can get the reference to the configuration drop-down in it's own event handler but if I try the exact same code from another drop-down's event handler it will be null
So something strange is going on. Is there another way to get a reference to that configuration drop-down in javascript? If I can get a handle to it somehow (even if not using the way you showed) then I can make this work
function onCategoryChange(e) {
var catDD = $("#Category").data("kendoDropDownList");
alert("cat is '" + catDD.value() + "'"); //displays correct value
var configDD = $("#Configuration").data("kendoDropDownList");
alert("config is '" + configDD.value() + "'"); //TypeError: configDD is undefined
}
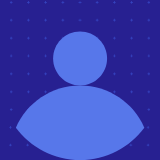
I think I see what's happening, if I view this in Firefox's console, the Inspector will display what is going on behind the scenes, I urge you to do the same so you can follow along.
These controls are only rendered as drop downs when they gain focus, you can see it's a script running that flips when the dropdown is clicked on to make a choice. When they do not have focus they are just rendering as a <span> tag with the text of their selected value displayed in the grid's cell.
That's why onCategoryChange(e) can get a handle to the Category drop down because it has focus but it cannot get a handle to the Configuration drop down because its not being rendered as a drop down at that point.
I confirmed this by doing the following. First I created an event handler for the Configuration drop-down like this:
var ddl = null;
function onConfigurationChanged(e) {
ddl = this;
}
then in the Category dropdown's change event I reference that global variable I set and it works as you would expect, but only if you first change the value of that Configuration drop down so that it sets the global variable
//this works fine until you move to another row and then you would need to 'reset'
//the ddl variable by triggering onConfigurationChange again
function onVariableCategoryChange(e) {
console.log(ddl.text());
console.log(ddl.value());
}
There's gotta be a solution to this, this is a common design for inline grid edits. Please help
Hello Scott,
The described is valid only if incell (batch) editing is used for the Grid. In this case when editing the Category field does not render the DropDownList for the Configuration field. If this is so I could suggest to conditionally disable the Configuration DropDownList on Edit event of the Grid:
function on Edit(e){
var configElem = e.container.find("[name='Configuration']");
if(e.model.Category == "NONE" && configElem.length) {
configElem.data("kendoDropDownList").enable(false);
}
}
Please try this and let me know if it helps.
In case the scenario is different it will help me a lot to investigate if you can prepare and share a sample runnable page that clearly replicates and isolates the problem. Examining this page locally will help me fully understand the case and eventually I will be able to provide a further solution.
Regards,
Nikolay
Progress Telerik
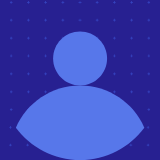
Yes, I actually figured this one out on my own just combing thru the code. I had set it to InCell by mistake
I'm going to post this in another thread but I will ask it here as well in case you can reply since it is the same grid as I described in the initial question. We got a request to convert the 2nd dropdown (Department) to be an editable combobox. I made the changes for that and I wrote a little ajax call to add the new item to the database if they enter a new free-form value. The problem I ran into is that even though it adds it correctly and the ajax call returns the newly added item with its new PK the grid row that gets submitted to the controller does not reflect this. Let me explain, here's my ajax call
//e is the Department combobox
function addDepartment(e) {
var dept = new Object();
dept.DepartmentID = 0;
dept.DepartmentName = e.text();
$.ajax(
{
url: '@Url.Action("AddDepartment", "Wizard")',
type: 'GET',
dataType: "json",
data: dept,
contentType: 'application/json; charset=utf-8',
success: function (result) {
console.log("success");
//re-fetch the datasource so the combo has the newly added item in it with its new PK
this.dataSource.read();
//I tried forcing its selection like this but it didn't help
e.value(result.DepartmentID);
}
})
console.log("variabletype added");
}
What happens here is that the new Dept is saved but the item selected in the combobox is not this new item. BUT if I select another item from the combo then re-select this newly added one, it will correctly be reflected in the save. Keep in mind these dropdowns are all using custom templates like in this demo: https://demos.telerik.com/aspnet-core/grid/editing-custom so the datasource has an object type Department that this is bound to.
What do I need to do to make this work?
Hello Scott,
I am glad to hear you managed to move forward with the project.
As we require all new requests to be submitted in separate threads I will close this one and will respond to the newly submitted ticket. This helps us track issues better and helps to provide faster response time.
Regards,
Nikolay
Progress Telerik
Our thoughts here at Progress are with those affected by the outbreak.