This is something that this grid has http://www.trirand.com/blog/jqgrid/jqgrid.html#
Thank you so much
7 Answers, 1 is accepted
Thank you for getting in touch with us.
In order to achieve that you should add search input inside the Grid's toolbar via template. When the value of this input changes you may filter the DataSource of the Grid using filter method of the DataSource.
Similar approach is demonstrated in this demo: http://demos.kendoui.com/web/grid/toolbar-template.html
Kind regards,
Alexander Valchev
the Telerik team
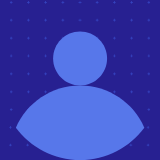
I found a way to do this.
First you create a toolbar with a input field on the Kendo grid like this:
@(Html.Kendo().Grid<
KdG.ProjectPortofolio.IntranetApp.ViewModels.Projects.ListProjectViewModel
>()
.ToolBar(toolBar => {
toolBar.Template(
@<
Text
>
<
input
type
=
"search"
id
=
"searchbox"
class
=
"SearchRight SearchTopMargin"
/>
<
b
class
=
"FloatRight SearchTopMarginExtra"
>Search the grid: </
b
>
</
Text
>);
}
<
script
type
=
"text/javascript"
>
$(document).ready(function () {
//change event
$("#searchbox").keyup(function () {
var val = $('#searchbox').val();
$("#Grid").data("kendoGrid").dataSource.filter({
logic: "or",
filters: [
{
field: "Name",
operator: "contains",
value: val
},
{
field: "Status",
operator: "contains",
value: val
},
{
field: "Category",
operator: "contains",
value:val
},
{
field: "AreaOfStudy",
operator: "contains",
value:val
},
]
});
});
});
</
script
>
I hope this info helps!
Regards,
Tim
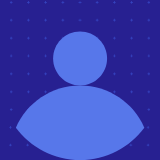
$(
"#category"
).keyup(
function
() {
var
selecteditem = $(
'#category'
).val();
var
kgrid = $(
"#grid"
).data(
"kendoGrid"
);
selecteditem = selecteditem.toUpperCase();
var
selectedArray = selecteditem.split(
" "
);
if
(selecteditem) {
//kgrid.dataSource.filter({ field: "UserName", operator: "eq", value: selecteditem });
var
orfilter = { logic:
"or"
, filters: [] };
var
andfilter = { logic:
"and"
, filters: [] };
$.each(selectedArray,
function
(i, v) {
if
(v.trim() ==
""
) {
}
else
{
$.each(selectedArray,
function
(i, v1) {
if
(v1.trim() ==
""
) {
}
else
{
orfilter.filters.push({ field:
"ProductName"
, operator:
"contains"
, value:v1 },
{ field:
"QuantityPerUnit"
, operator:
"contains"
, value:v1});
andfilter.filters.push(orfilter);
orfilter = { logic:
"or"
, filters: [] };
}
});
}
});
kgrid.dataSource.filter(andfilter);
}
else
{
kgrid.dataSource.filter({});
}
});
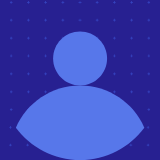
I know this is a old post. But i wanted to provide this for anyone coming back here. I needed something a little more generic, This takes all string fields in the grid and searchs against them. I put this function in a global js file and now anytime a grid is loaded on the screen it dynamically adds a search box and searches all string fields on the grid. Thanks @Tim for the initial code which I based my solution off of.
//Global Grid Search
$(document).ready(
function
() {
//change event
$(
'.k-grid-add'
).after(
'<input type="search" id="searchbox" class="k-autocomplete k-input pull-right" placeholder="Search...">'
);
$(
"#searchbox"
).keyup(
function
() {
var
val = $(
'#searchbox'
).val();
var
grid = $(
"#grid"
).data(
"kendoGrid"
);
if
(val) {
var
filters = []
$.each(grid.options.dataSource.fields,
function
(index, value) {
if
(value.field && grid.options.dataSource.schema.model.fields[value.field].type ==
"string"
) {
var
filter = {
field: value.field,
operator:
"contains"
,
value: val
}
filters.push(filter);
}
})
$(
"#grid"
).data(
"kendoGrid"
).dataSource.filter({
logic:
"or"
,
filters: filters
});
}
else
{
$(
"#grid"
).data(
"kendoGrid"
).dataSource.filter({
logic:
"or"
,
filters: []
});
}
});
});
Please note that I use kendo mvc scaffolder and it names all grids "grid" by default and not "Grid" as shown in @Tim's code.
You may need to update the line: var grid = $("#grid").data("kendoGrid"); to match your grid name.
Since I use JS to inject the search box you do not need to make any changes to your .cshtml which is very nice and generic.
CSS Below if you want it:
#searchbox {
height
:
25px
;
margin-right
:
15px
;
padding-left
:
5px
;
}
Forgot to mention you need to have the default "Add new record" button on the toolbar as thats how I inject the search bar. If you don't have a search bar then change line 4 to something more appropriate.
Here is the default cshtml toolbar i'm attaching to (line 9-11)...
01.
@(Html.Kendo().Grid<
SecurityAccessApplication.Models.State
>()
02.
.Name("grid")
03.
.Columns(columns =>
04.
{
05.
columns.Bound(c => c.Abbreviation);
06.
columns.Bound(c => c.Name);
07.
columns.Command(command => { command.Edit(); }).Width(180);
08.
})
09.
.ToolBar(toolbar => {
10.
toolbar.Create();
11.
})
12.
.Editable(editable => editable.Mode(GridEditMode.InLine))
13.
.Pageable()
14.
.Filterable()
15.
.Scrollable()
16.
.DataSource(dataSource => dataSource
17.
.Ajax()
18.
.Model(model => model.Id(p => p.ID))
19.
.Read(read => read.Action("States_Read", "State"))
20.
.Create(create => create.Action("States_Create", "State"))
21.
.Update(update => update.Action("States_Update", "State"))
22.
)
23.
)
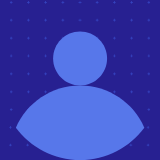
Apologies for resurrecting this thread, but it's the first result for this question on Google and thought I'd share.
I combined the solutions by Thomas and Dhruv to be able to search like in Jquery datatables on all string fields.
// When typing on the search box
$(
'.searchStringInput'
).keyup(function () {
var table = $(
"#grid"
).data(
"kendoGrid"
);
var searchText = $(
this
).val().toUpperCase();
var searchTextArray = searchText.split(
" "
);
if
(searchText) {
var orfilter = { logic:
"or"
, filters: [] };
var andfilter = { logic:
"and"
, filters: [] };
$.each(searchTextArray, function (i, txt1) {
if
(txt1.trim() ==
""
) {
}
else
{
$.each(searchTextArray, function (i, txt2) {
if
(txt2.trim() ==
""
) {
}
else
{
$.each(table.options.dataSource.options.schema.model.fields, function (index, value) {
if
(value.field && table.options.dataSource.options.schema.model.fields[value.field].type ==
"string"
) {
var filter = {
field: value.field,
operator
:
"contains"
,
value: txt2
}
orfilter.filters.push(filter);
}
});
//each
andfilter.filters.push(orfilter);
orfilter = { logic:
"or"
, filters: [] };
}
});
//each
}
});
// each
table.dataSource.filter(andfilter);
}
else
{
table.dataSource.filter({});
}
});
Thank you very much for the provided solution.
If you are not using serverfiltering, you can filter the entire grid for numbers, dates, strings and booleans as shown in this how-to article rom our knowledge base:
https://docs.telerik.com/kendo-ui/knowledge-base/filter-all-columns-with-one-textbox
In addition to that, I am pleased to let you know this has been a very popular feature request and we have created it as a toolbar feature. It is due to be released in R3 scheduled or 18th September 2019.
Kind Regards,
Alex Hajigeorgieva
Progress Telerik
Hello, Amrish,
It is not very clear if you are using the built-in search panel or the knowledge base article that we have discussed:
https://demos.telerik.com/kendo-ui/grid/search-panel
If you are using the built-in search panel over two fields, can you use the API live example to show us the behaviour you are seeing? You can click on the Open In Dojo link and add the sample fields and data and send us the Dojo url:
https://docs.telerik.com/kendo-ui/api/javascript/ui/grid/configuration/search.fields
Look forward to hearing back from you.
Kind Regards,
Alex Hajigeorgieva
Progress Telerik
Our thoughts here at Progress are with those affected by the outbreak.
HI,
I'm facing issue , when search anything, searched content disappear with in second.
I'm applying search on two columns, one code and second one title.
title column search is working fine, but when text matches to Code column value, text disappeared .