I was wondering if someone could explain to me how to get the datakeyvalue of a selected row on the server side and outside of a radgrid event.
For instance say I have a radgrid and it has a row that has been selected. When someone clicks a button (called btnTest for instance) that is somewhere else on the page, how do I go about getting the datakeyvalue of the selected row in btnTest’s click event.
15 Answers, 1 is accepted

Try the following code snippet to achieve the desired scenario.
ASPX:
<ClientSettings > |
<Selecting AllowRowSelect="true" /> |
</ClientSettings> |
<MasterTableView DataKeyNames="CustomerID" > |
CS:
protected void Button1_Click(object sender, EventArgs e) |
{ |
foreach (GridDataItem item in RadGrid1.MasterTableView.Items) |
{ |
if (item.Selected) |
{ |
string strKey = item.GetDataKeyValue("CustomerID").ToString(); |
} |
} |
} |
Thanks
Shinu.
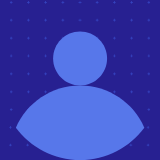
I have already found this snippet in the help file:
var firstDataItem = $find("<%= rgGrid1.ClientID %>").get_masterTableView().get_dataItems()[0];
var keyValues = firstDataItem.getDataKeyValue("unique_id");
This does not get SELECTED row's data key, only first row.
I need to get this outside of a radgrid client-side event as well.
thanks.
Please examine the approach shown below:
function buttonClick() |
{ |
var radgrid = $find('<%= RadGrid1.ClientID %>'); |
alert(radgrid.get_masterTableView().get_selectedItems()[0].getDataKeyValue("OrderID")); |
} |
Kind regards,
Daniel
the Telerik team
Check out Telerik Trainer, the state of the art learning tool for Telerik products.
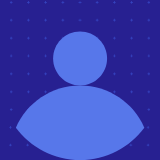
I have a grid and 3 linkbuttons inside it then i want to trigger seperate events when each of the butttons are clicked not when the row is selected.
I have been able to get the buttons respond on sepearate clicks but i could retrieve any value from that row neither could I acces the clientdatakeynames of the grid
I will be glad to have a response
waiting sirs ...
Thanks in anticipation

<
MasterTableView
DataKeyNames
=
"ID"
>
protected
void
btn1_Click(
object
sender, EventArgs e)
{
Button btn1 = sender
as
Button;
GridDataItem item = btn1.NamingContainer
as
GridDataItem;
string
ID = item.GetDataKeyValue(
"ID"
).ToString();
}
Let me know if any concern.
Thanks,
Jayesh Goyani

You can try the following method to access ClientDataKeyValues in onclick event of LinkButton.
C#:
protected
void
RadGrid1_ItemDataBound(
object
sender, GridItemEventArgs e)
{
if
(e.Item
is
GridDataItem)
{
GridDataItem item = (GridDataItem)e.Item;
LinkButton link = (LinkButton)item.FindControl(
"LinkButton1"
);
int
rowindex = item.ItemIndex;
link.Attributes.Add(
"onclick"
,
"clicked('"
+ rowindex +
"');"
);
}
}
Javascript:
function
clicked(rowindex)
{
var
row = $find(
'<%= RadGrid1.ClientID %>'
).get_masterTableView().get_dataItems()[rowindex];
alert(row.getDataKeyValue(
"ID"
));
//DataKeyName
}
Thanks,
Princy.
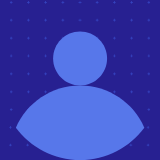
This Fantastic Code just bailed me out
protected void getAcceptRequest_Button_Click(object sender, System.EventArgs e)
{
LinkButton butAccept = (LinkButton)sender;
GridDataItem editItem = (GridDataItem)butAccept.NamingContainer;
HiddenField hfRequest_Id = (HiddenField)editItem.FindControl("hfRequestId");
string requestId = hfRequest_Id.Value;
HiddenField hfRequest_From = (HiddenField)editItem.FindControl("hfRequestFrom");
string requestFrom = hfRequest_From.Value;
HiddenField hfRequest_To = (HiddenField)editItem.FindControl("hfRequestTo");
string requestTo = hfRequest_To.Value;
runAccept(requestId, requestFrom, requestTo);
}
I appreciate!

Is that possible to add multiple datakeys on a Rad Grid.. please give the code
Regards,
Prassin

Please try setting the DataKeyNames property of the MasterTableView as given below.
ASPX:
<
telerik:RadGrid
ID
=
"Rad"
runat
=
"server"
AllowFilteringByColumn
=
"true"
DataSourceID
=
"SqlDataSource1"
AutoGenerateColumns
=
"false"
>
<
MasterTableView
DataKeyNames
=
"OrderID,ShipName"
>
. . .
</
MasterTableView
>
Thanks,
Shinu.
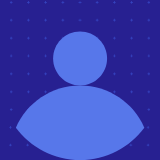
Thank you Daniel. Very useful. Worked for me.
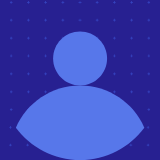
Object reference not set to an instance of an object.
what should i do now ??

Can you please elaborate your scenario / provide you code?
Thanks,
Jayesh Goyani

I couldn't get the DataKeyValue at client side using RoeIndex.
var r=$find('<%= Rg_Centers.ClientID%>').get_masterTableView().get_dataItems()[index];
alert(r.getDataKeyValue("CenterId"));
the above code gives null value.

Please make sure you have set the ClientDataKeyNames for the Grid.
ASPX:
<
MasterTableView
ClientDataKeyNames
=
"CenterId"
DataKeyNames
=
"CenterId"
>
Thanks,
Princy

Its working fine.
Can anyone help me out how to assign row.KeyValues["TestGuid"] to TestGuid.Value.It is not working with migrated RadGrid to Asp.Net Ajax.
After migrating from Classic RadControls ,It stopped working and throwing error , can anyone help me what should be replaced in that case.
Here is the Code ,
<div style="display:none">
<asp:Button ID="btnSelect" CausesValidation="false" runat="server" OnClick = "btnSelect_Click" />
<input type="hidden" id="hidType" name="hidType" runat="server" />
<input type="hidden" id="hidGuid" name="hidGuid" runat="server" />
<asp:Button ID="btnRemove" CausesValidation="false" runat="server" OnClick = "btnRemove_Click" />
<input type="hidden" id="hidCheck" name="hidCheck" runat="server" />
<input type="hidden" id="hidRemove" name="hidRemove" runat="server" />
</div>
Javascript Code for the selected Row for RadGrid:
function TestItemRowSelected(row)
{
var TestGuid = document.getElementById('<%= hidGuid.ClientID %>');
var showType = document.getElementById('<%= hidType.ClientID %>');
TestGuid.value = row.KeyValues["TestGuid"]; ---->Issue
showType.value = "Test";
var oButton = document.getElementById("<%=btnSelect.ClientID%>");
oButton.click();
}
Error :
Uncaught TypeError: row.KeyValues is not a functionat Array.TestItemRowSelected (StaticShell.aspx?Portlet=ConnectReports:264)
at ScriptResource.axd?d
at Telerik.Web.UI.RadGrid.raiseEvent
at Telerik.Web.UI.RadGrid.raise_rowSelected (
at Telerik.Web.UI.GridSelection._selectRowInternal
at Telerik.Web.UI.GridSelection._click
at HTMLDivElement.<anonymous>
at HTMLDivElement.browserHandler