I have a form generated using Html.Kendo().Form. I would like to be able to export the contents of the form (labels, textboxes, checkboxes) to a new PDF on the click of a button, and then subsequently merge it with another PDF.
Is there a generic method that would enable me to do this, rather than using the PdfProcessing Library to create a new document and then add each of the fields from the ViewModel one by one.
Thank you.
2 Answers, 1 is accepted
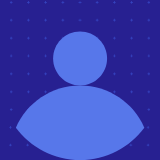
I eventually got the import from URL to work - there was a hidden error about being unable to find the style sheet, so I included a HtmlImportSettings property:
// Create a document instance from the content
HtmlFormatProvider provider = new HtmlFormatProvider();
HtmlImportSettings importSettings = new HtmlImportSettings();
importSettings.LoadStyleSheetFromUri += (s, e) =>
{
string styles = System.IO.File.ReadAllText(@"wwwroot\" + e.Uri);
e.SetStyleSheetContent(styles);
};
provider.ImportSettings = importSettings;
RadFlowDocument headerDocument = null;
using (WebClient webClient = new WebClient())
{
var response = webClient.DownloadData(url);
headerDocument = provider.Import(new MemoryStream(response));
}
PdfFormatProvider pdfProvider = new PdfFormatProvider();
// Export the document
Stream headerStream = new MemoryStream(pdfProvider.Export(headerDocument));
I was then able to merge the headerStream with a second PDF using a PdfStreamWriter.
Richard
Hello Richard,
Thank you for writing to us.
This requirement is possible to achieve theoretically and this functionality is demonstrated in Implementation Section point 5 and point 6 of this blog post:
https://www.telerik.com/blogs/editing-pdf-files-in-browser-adding-signature-text-images
Feel free to check it and let me know if this sample helps you.
Regards,
Eyup
Progress Telerik
Virtual Classroom, the free self-paced technical training that gets you up to speed with Telerik and Kendo UI products quickly just got a fresh new look + new and improved content including a brand new Blazor course! Check it out at https://learn.telerik.com/.
Hello Eyup,
Thank you for your reply.
I was wondering if there is a way to Export a page to PDF in the controller itself? For instance, by using a url to get a response from a webpage I can get the raw html (responseStr) using a StreamReader. However, using a html provider and RadFlowDocument I can't see to Import the response - the RadFlowDocument is blank in the example below.
var request = HttpWebRequest.Create(url);
string responseStr = string.Empty;
HtmlFormatProvider htmlProvider = new HtmlFormatProvider();
RadFlowDocument headerDocument = new RadFlowDocument();
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
using (var responseStream = new StreamReader(response.GetResponseStream()))
{
responseStr = responseStream.ReadToEnd();
}
// Or
using (Stream input = response.GetResponseStream())
{
headerDocument = htmlProvider.Import(input);
}
}
Once I've got the first document (created from the imported html) I want to merge it with another PDF.
Is there a way to generate the pdf from the Web Response using the Telerik PDF processing libraries?
Kind regards,
Richard
This live sample, albeit for another technology, demonstrates that PDF files can be processed using Streams:
https://demos.telerik.com/aspnet-ajax/pdfviewer/applicationscenarios/dplintegration/defaultcs.aspx
Make sure that the stream is complete and the PDF file is properly parsed.
Hi Eyup,
Many thanks again for your reply. The example that you included is close to something that I was working on. I think the difficulty is getting the complete stream. So far I've got the following, but it gives me a null document based on a url to the web page that I want in the document. Any ideas what I've done wrong?
var request = HttpWebRequest.Create(url);
// Create a document instance from the content
IFormatProvider<RadFlowDocument> provider = new HtmlFormatProvider();
RadFlowDocument document = null;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
document = provider.Import(response.GetResponseStream());
}
Richard
demos.telerik.com/aspnet-core/pdf-export converts the DOM element (my form) to a drawing using kendo.drawing.drawDOM, and then uses kendo.drawing.exportPDF to render the result as a PDF file.
I need the base64 contents to merge with another PDF document using the PdfStreamWriter before writing the finished File.
Can the DOM element be passed to a Controller method so that the Kendo Drawing methods can be used, and then I can merge it with the PDF?