8 Answers, 1 is accepted
Thank you for writing.
The DefaultValueAttribute defines the default value to which the property will be reset. When the property value is set to something different the default value, it will be marked as modified. Here is a sample code snippet demonstrating how to use the default value:
public
RadForm1()
{
InitializeComponent();
this
.radPropertyGrid1.SelectedObject =
new
Item();
}
public
class
Item
{
[DefaultValue(5)]
public
int
Id {
get
;
set
; }
[DefaultValue(
"Test"
)]
public
string
Name {
get
;
set
; }
[DefaultValue(
typeof
(Color),
"Red"
)]
public
Color BackColor {
get
;
set
; }
public
Item()
{
this
.Id = 5;
this
.Name =
"Empty"
;
this
.BackColor = Color.Red;
}
}
I hope this information helps. Should you have further questions I would be glad to help.
Regards,
Dess
Progress Telerik
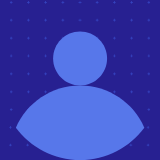
public byte[] HiRgb = { 128, 128, 128 };
[DefaultValue(typeof(Color), "Gray")]
public Color HiColor
{
get => Color.FromArgb(HiRgb[0], HiRgb[1], HiRgb[2]);
set
{
HiRgb[0] = value.R; HiRgb[1] = value.G; HiRgb[2] = value.B;
}
}
This always marked as modified. Why?
Thank you for writing back.
Each value that differs from the default value is marked as edited. Note that if you compare Color.Gray and Color.FromArgb(128,128,128) the obtained result will be false. That is why the HiColor is marked as modified. You can set the HiColor value to Color.Gray. Thus, it won't be bold.
I hope this information helps. If you have any additional questions, please let me know.
Regards,
Dess
Progress Telerik
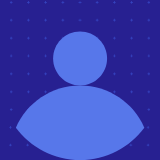
Ok.
How can I change default values during runtime?
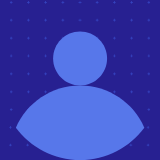
PS.
In winforms I use ShouldSerialize and Reset Methods
https://docs.microsoft.com/en-us/dotnet/framework/winforms/controls/defining-default-values-with-the-shouldserialize-and-reset-methods
Is there an analog in your program?
Thank you for writing back.
Note that the metadata in DefaultValueAttribute is retrieved when it is first requested and it is cached for subsequent retrieval. In order to change the attribute value for a specific property at runtime, you can directly modify the property descriptor for the given property. Here is a code snippet demonstrating this:
AttributeCollection attributes = TypeDescriptor.GetProperties(
this
.radPropertyGrid1.SelectedObject)[
"HiColor"
].Attributes;
DefaultValueAttribute myAttribute = (DefaultValueAttribute)attributes[
typeof
(DefaultValueAttribute)];
FieldInfo defaultValueField = (
typeof
(DefaultValueAttribute)).GetField(
"value"
, BindingFlags.Instance | BindingFlags.NonPublic);
defaultValueField.SetValue(myAttribute, Color.Fuchsia);
RadPropertyGrid offers to reset a property to its default value by using the default context menu:
I hope this information helps. If you have any additional questions, please let me know.
Regards,
Dess
Progress Telerik
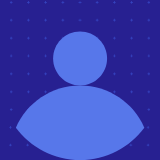
I do not need to reset a property to its default value.
I need change default value during runtime. For example, if another property has changed the state machine and i need a different default color.
use of reflection is inconvenient
Thank you for writing back.
Changing attributes' values is not a usual requirement for RadPropertyGrid users. As it was previously explained, the metadata in DefaultValueAttribute is retrieved when it is first requested and it is cached for subsequent retrieval. It is not expected to be further modified. Currently, the possible solution that I can suggest to use reflection as it is demonstrated in the previous reply.
I hope this information helps. If you have any additional questions, please let me know.
Regards,
Dess
Progress Telerik