I was wondering if any one had a working example of loading data onto a grid using a Java action?
The following is a code snippet of something I did a while back using DHTMLX Grid and Dojo.
So, inside my JSP I call the Java action that returns the data in XML format like so:
<
script
>
var mysubgrid;
mysubgrid = new dhtmlXGridObject('gridbox');
function doInitSubGrid(rowID)
{
var leadsSourceRecId = rowID ;
var URL = "" ;
var query = "pLeadsSourceRecId=" + leadsSourceRecId ;
mysubgrid = new dhtmlXGridObject('mysubgrid_container');
mysubgrid.setImagePath("../dhx/codebase/imgs/");
mysubgrid.setHeader("LEADS MASTER LIST,#cspan,#cspan,#cspan,#cspan,#cspan") ;
mysubgrid.attachHeader("Status, Source, Date, Name, Email, Home Phone");
mysubgrid.attachHeader("#text_filter,#text_filter,#text_filter,#text_filter,#text_filter,#text_filter");
mysubgrid.setInitWidths("80,100,100,170,170,100");
//mysubgrid.setColAlign("left,left,left,left,left,left")
mysubgrid.setSkin("light");
mysubgrid.setColSorting("str,str,str,str,str,str");
mysubgrid.setColTypes("ro,ro,ro,ro,ro,ro");
mysubgrid.attachEvent("onRowSelect",doOnRowSelectedSubGrid);
mysubgrid.init();
// HERE IS WHERE I CALL MY JAVA ACTION: GetLeadsGridDataStr.action
// I PASS A PARAMETER CALLED pLeadsSourceId
mysubgrid.loadXML("GetLeadsGridDataStr.action?pLeadsSourceRecId=" + leadsSourceRecId) ;
}
</
script
>
The data is then rendered on the DHTMLX Grid.
Does Anybody have an example like this one using Kendo?
16 Answers, 1 is accepted
The Grid widget uses a DataSource component in order to retrieve and manipulate data. The DataSource is responsible to retrieve the data from local/remote source. You can find more details on what DataSource is and how it operates in the following articles:
http://www.kendoui.com/documentation/framework/datasource/overview.aspx
http://demos.kendoui.com/web/datasource/remote-data.html
To your question - you need to setup the dataSource of the Grid in order to retrieve data from the view. The view on the other hand must return a valid JSON.
http://demos.kendoui.com/web/grid/remote-data.html
That all you need in order to provide the data from any server technology.
Best wishes,
Nikolay Rusev
the Telerik team
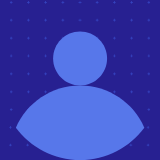
Hi Nikolay,
I’m working with one of the examples provided by the KendoUI download to try to get it to work with Java.
I create a Java class called: GetDataGrid.class
If invoked through the URL, this Java class returns the following string:
([{"ProductID":1,"ProductName":"Chai","UnitPrice":18,"UnitsInStock":39,"Discontinued":false},{"ProductID":2,"ProductName":"Chang","UnitPrice":19,"UnitsInStock":17,"Discontinued":false},{"ProductID":3,"ProductName":"Aniseed Syrup","UnitPrice":10,"UnitsInStock":13,"Discontinued":false}])
I then modified the JavaScript and replaced /Products with /GetDataGrid.action.
<
script
>
$(document).ready(function () {
var crudServiceBaseUrl = "",
dataSource = new kendo.data.DataSource({
transport: {
read: {
// Modified from /Products to /GetDataGrid.action
url: crudServiceBaseUrl + "/GetDataGrid.action",
dataType: "jsonp"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function(options, operation) {
if (operation !== "read" && options.models) {
return {models: kendo.stringify(options.models)};
}
}
},
batch: true,
pageSize: 30,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true } }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
navigatable: true,
pageable: true,
height: 400,
toolbar: ["create", "save", "cancel"],
columns: [
"ProductName",
{ field: "UnitPrice", format: "{0:c}", width: "150px" },
{ field: "UnitsInStock", width: "150px" },
{ field: "Discontinued", width: "100px" },
{ command: "destroy", title: " ", width: "110px" }],
editable: true
});
});
</
script
>
However, when I run the HTML page the data does not load on the grid.
What am I doing wrong?
As the response is in the same domain and is JSON not JSONP you will have to remove the dataType: "jsonp" in order to bind the Grid.
The response seems to be a valid JSON so it should bind to the Grid.
For your convenience I am attaching sample app built with Play framework that demonstrates how to bind Kendo Grid. The view resides in /app/views/application/index.html
and the controller in /app/controllers/Application.java
Regards,
Nikolay Rusev
the Telerik team
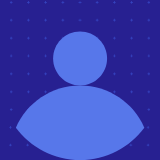
Hi Nikolay,
Got it working – thank you.
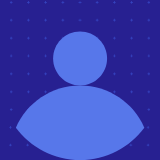
Ok, I’m posting this for anyone using Java EE to build the server side components.
I used the following code to populate a data grid:
So, my HTML page contains the following:
<
script
>
$(document).ready(function () {
var crudServiceBaseUrl = "/site",
dataSource = new kendo.data.DataSource({
transport: {
read: {
url: crudServiceBaseUrl + "/GetDataGrid.action",
dataType: "json"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function(options, operation) {
if (operation !== "read" && options.models) {
return {models: kendo.stringify(options.models)};
}
}
},
batch: true,
pageSize: 30,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true } }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
navigatable: true,
pageable: true,
height: 400,
toolbar: ["create", "save", "cancel"],
columns: [
"ProductName",
{ field: "UnitPrice", format: "{0:c}", width: "150px" },
{ field: "UnitsInStock", width: "150px" },
{ field: "Discontinued", width: "100px" },
{ command: "destroy", title: " ", width: "110px" }],
editable: true
});
});
</
script
>
My Java class (GetDataGrid .java), contains the following:
package com.kendoui;
import org.mentawai.core.*;
import org.mentawai.ajax.*;
import org.json.JSONObject;
public class GetDataGrid extends BaseAction
{
// ______________________________________________________
// START DECLARE NECESSARY FIELDS
// END DECLARE NECESSARY FIELDS
// ______________________________________________________
public String execute() throws Exception
{
StringBuffer jsonBuf = new StringBuffer() ;
JSONObject jsonObj = new JSONObject();
jsonObj.put("ProductID",new Integer(3));
jsonObj.put("ProductName","Toshiba");
jsonObj.put("UnitPrice",new Double(255.00));
jsonObj.put("UnitsInStock",new Integer(100));
jsonObj.put("Discontinued",false);
jsonBuf.append(jsonObj.toString()) ;
jsonObj.isNull(null) ;
jsonObj.put("ProductID",new Integer(100));
jsonObj.put("ProductName","Acer");
jsonObj.put("UnitPrice",new Double(300.00));
jsonObj.put("UnitsInStock",new Integer(13));
jsonObj.put("Discontinued",false);
jsonBuf.append(",").append(jsonObj.toString()) ;
jsonObj.isNull(null) ;
jsonObj.put("ProductID",new Integer(88));
jsonObj.put("ProductName","Dell");
jsonObj.put("UnitPrice",new Double(475.00));
jsonObj.put("UnitsInStock",new Integer(135));
jsonObj.put("Discontinued",false);
jsonBuf.append(",").append(jsonObj.toString()) ;
output.setValue(AjaxConsequence.OBJECT, "[" + jsonBuf.toString() + "]");
return SUCCESS;
}
}
Enjoy.
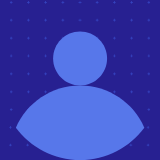
output.setValue(AjaxConsequence.OBJECT, "[" + jsonBuf.toString() + "]");
Could u help me to clear the error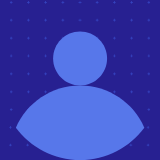
I am working on the kendo grid. i am trying to fetch the data from database using java, I got the value on the java , bot it does not print the value in grid. I am using the PrintWriter to print the values and my code and the java result are
<
div
id
=
"movies-container"
class
=
"k-widget k-header k-menu-vertical"
>
<
ul
id
=
"movies"
></
ul
>
</
div
>
<
script
>
jQuery(document).ready(function(){
var dataSource = new kendo.data.DataSource({
transport: {
read:{
url:'ajaxClientload.action',
dataType: "json"
}
},
batch: true,
pageSize: 30,
schema:{
model:{
fields:{
FirstName:{type:"string"}
}
}
}
});
dataSource.read();
$("#movies").kendoGrid({
dataSource: dataSource,
navigatable: true,
pageable: true,
height: 400,
toolbar: ["create", "save", "cancel"],
columns: [
{ field: "FirstName", width: "150px" }],
editable: true
});
});
</
script
>
{
"FirstName"
:
"James"
}
All you need to bind the Grid is to return valid JSON from server, i.e from 'ajaxClientload.action'.
All the best,
Nikolay Rusev
the Telerik team
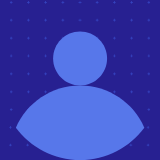
Thanks for your reply. The server returns the valid json, I don't know what i am missing i send a code to you, Can u help me
public String execute() throws Exception
{
StringBuffer jsonbuff = new StringBuffer();
JSONObject jsonObj = new JSONObject();
jsonObj.put("FirstName", "James");
print(jsonObj.toString());
System.out.println("sample " + jsonObj.toString());
return SUCCESS;
}
private boolean printed;
private PrintWriter out;
protected void print(String text)
{
printed = true;
getResponse().setContentType("application/json");
getOut().print(String.valueOf(text));
}
public PrintWriter getOut()
{
if(out == null)
{
try
{
setOut(getResponse().getWriter());
}
catch(IOException e)
{
// LogHandler.error(this, e);
}
}
return out;
}
The data that is returned must ba e collection. I see a single item returned from your action method (
{
"FirstName"
:
"James"
}
).All the best,
Nikolay Rusev
the Telerik team
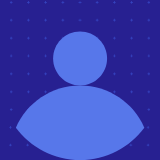
Thanks for ur reply. I got it it works fine.
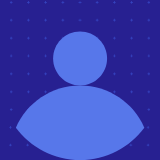
You did not specify the error you were getting but I have an idea of what it might be.
In my Java app, I’m using the Menta MVC. You’ll notice the following packages:
import org.mentawai.core.*;
import org.mentawai.ajax.*;
So, if you use the following in your Java class you will get an error:
output.setValue(AjaxConsequence.OBJECT, "[" + jsonBuf.toString() + "]");
You must either use the Menta API, look here:
Or return your value differently.
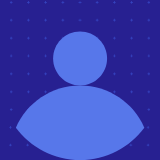
Thanks for your reply and code , It's working fine.
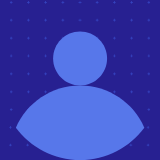
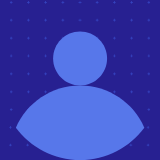
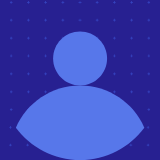
I can catch the event in java, but I can't find record ID